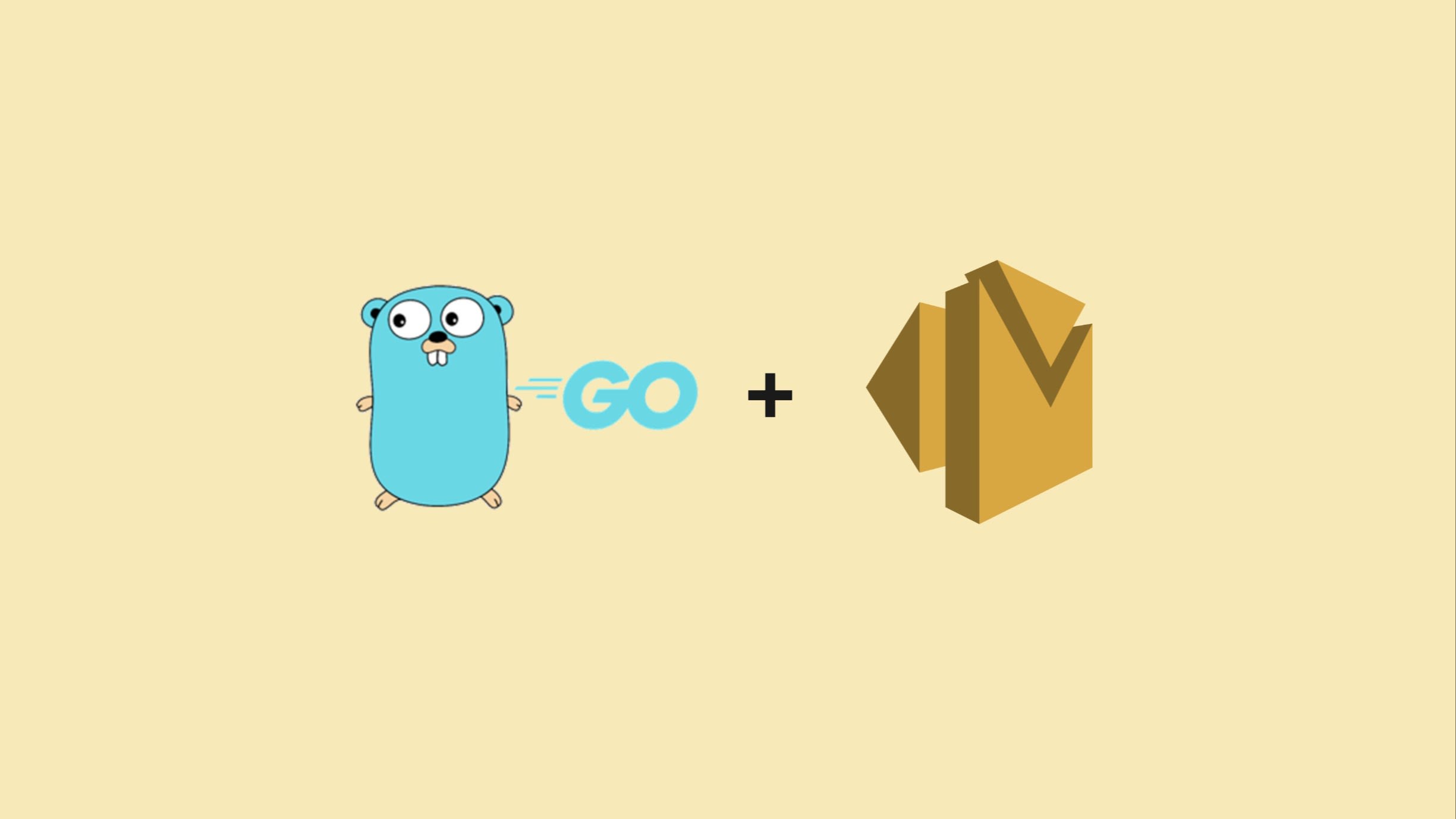
Home > Resources > All Blog posts
Simplifying Email Automation: How to Send Emails via AWS SES Using Golang
Semplates
Go
Golang
AWS SES
AWS SES SDK
AWS Simple Email Service
- Updated
5 min read
Dive into our latest guide where we unravel the intricacies of using Golang to seamlessly integrate with Amazon Simple Email Service (SES). Discover practical coding insights and streamline your email automation with confidence.
Any question?
Feel free to contact us anytime if you have any questions.
Return to all blog postsOn this page