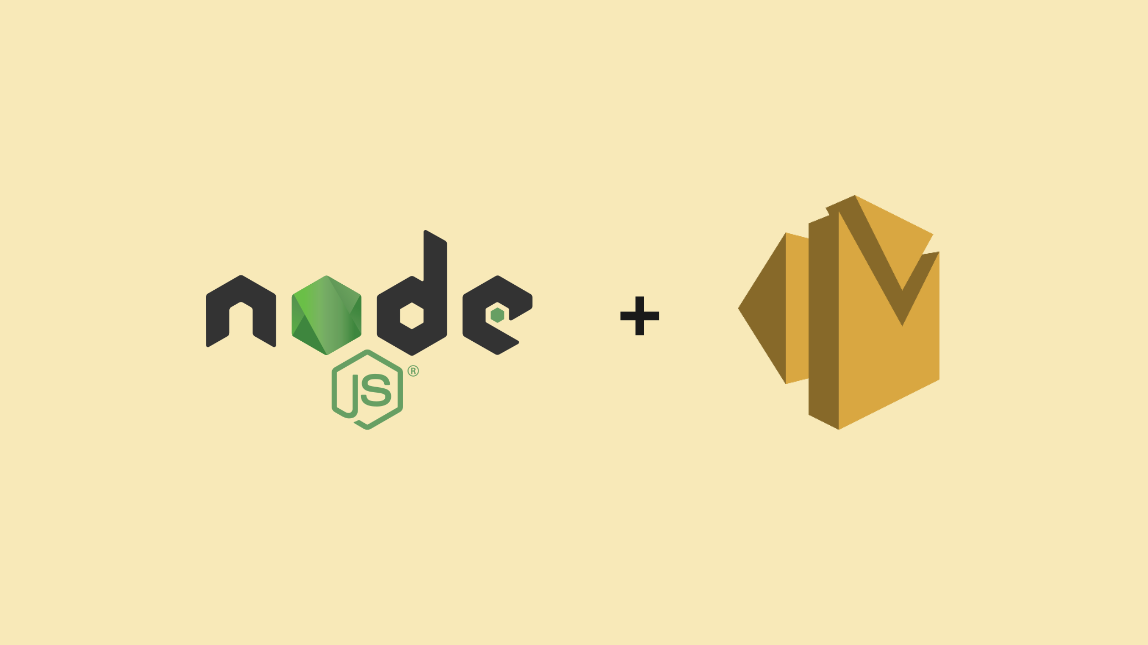
Home > Resources > All Blog posts
Sending Emails with Amazon SES Using Node.js: A Comprehensive Guide
Semplates
AWS SES
Node.js
Amazon SES SDK
Email Sending
Email Templates
Node.js Email
- Updated
5 min read
Uncover the step-by-step process of sending emails with Amazon SES using Node.js. Learn how to leverage the Node.js SDK for simple to complex API calls and explore how Semplates can enhance your template management.
Any question?
Feel free to contact us anytime if you have any questions.
Return to all blog postsOn this page