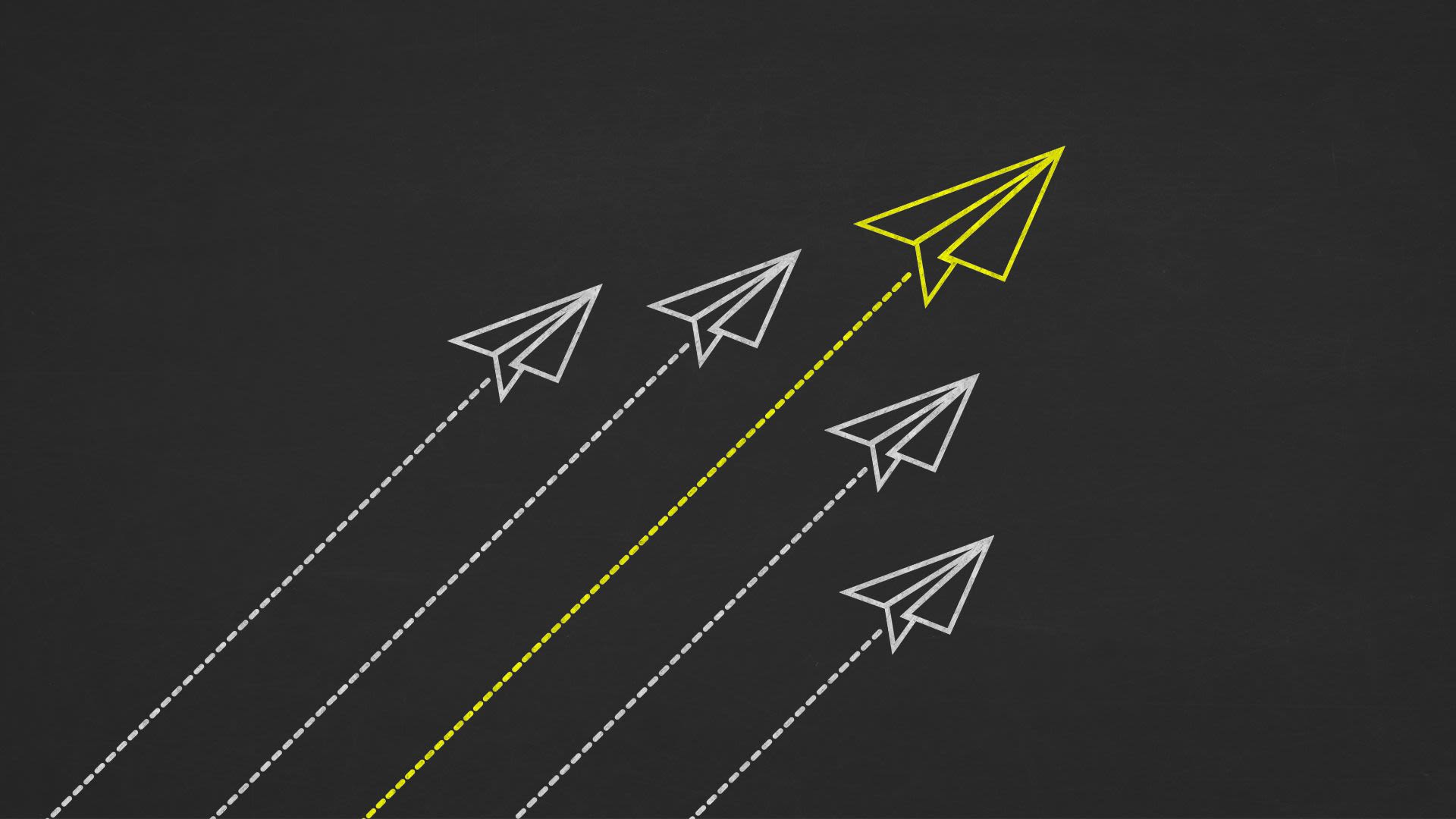
Home > Resources > All Blog posts
Mastering Email Delivery with Nodemailer and AWS SES
Semplates
Nodemailer
AWS SES
Email Templates
Node.js
Email Sending
Amazon SES
- Updated
2 min read
Learn how to efficiently send templated emails using Nodemailer and AWS SES. Discover the power of Semplates for easy and professional template management.
Any question?
Feel free to contact us anytime if you have any questions.
Return to all blog postsOn this page