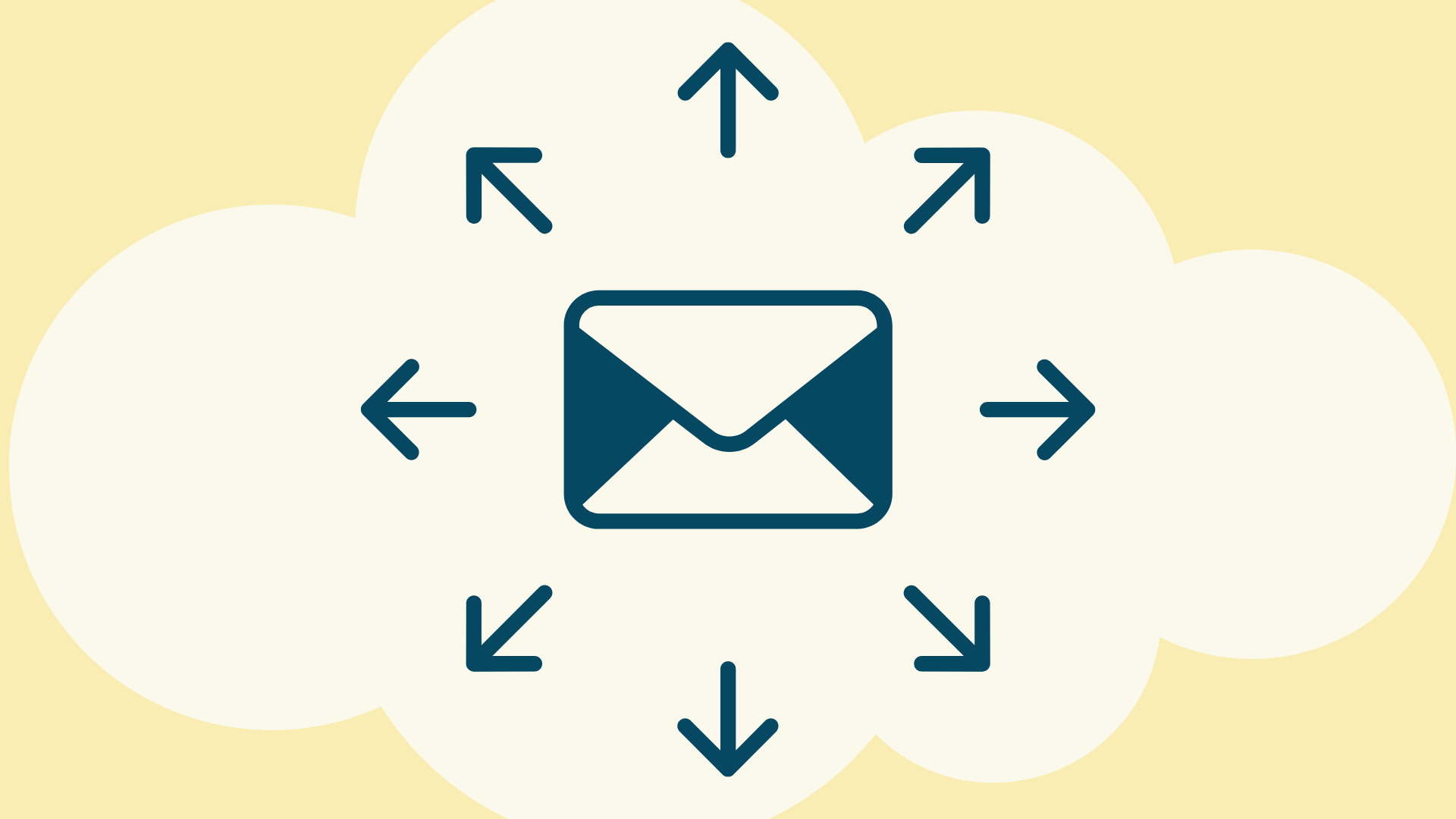
Home > Resources > All Blog posts
Maximizing Efficiency: AWS SES for Bulk Email Sending
Semplates
Bulk Email Sending
aws ses bulk email
Email Automation
sendBulkEmail
Send Bulk Email
- Updated
6 min read
Discover how to leverage AWS SES for bulk email sending, including tips on overcoming the 50-recipient limit and utilizing Semplates for easy, efficient bulk mailing. Perfect for businesses looking to scale their email marketing efforts.
Any question?
Feel free to contact us anytime if you have any questions.
Return to all blog postsOn this page